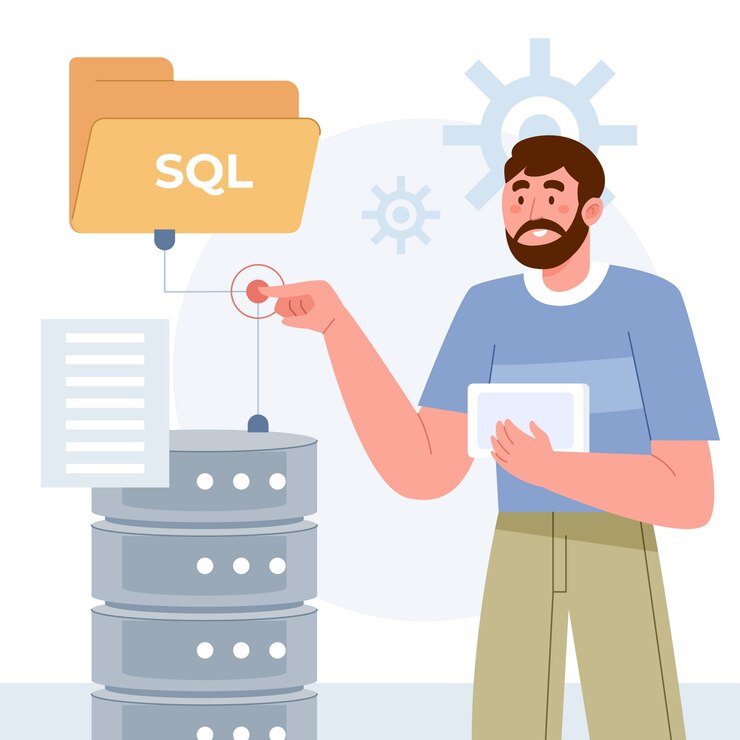
Introduction:
Structured Query Language, commonly known as SQL, is the cornerstone of database management systems. Its simplicity, power, and versatility have made it the industry standard for querying and managing databases. Whether you’re a seasoned developer, a data analyst, or someone new to the tech world, understanding SQL is essential. This article delves into the fundamentals of SQL, its core functionalities, and its significance in today’s data-driven landscape.
What is SQL?
SQL is a domain-specific language used in programming and managing relational databases. It was initially developed in the 1970s at IBM and has since become the standard language for relational database management systems (RDBMS). SQL’s primary functions include:
- Querying Data: Retrieving specific data from databases.
- Inserting Data: Adding new data into databases.
- Updating Data: Modifying existing data.
- Deleting Data: Removing data from databases.
- Creating and Modifying Database Structures: Defining and altering database schemas.
Core Components of SQL
Data Definition Language (DDL)
DDL commands are used to define the structure of the database, including creating, altering, and dropping tables and indexes. Key DDL commands include:
- CREATE: Creates a new table, index, or database.
CREATE TABLE Employees (
EmployeeID int,
FirstName varchar(255),
LastName varchar(255),
BirthDate date
);
- ALTER: Modifies an existing database object, such as a table.
ALTER TABLE Employees
ADD Email varchar(255);
- DROP: Deletes an existing database object.
DROP TABLE Employees;
Data Manipulation Language (DML)
DML commands are used for managing data within schema objects. These commands include:
- SELECT: Retrieves data from the database.
SELECT FirstName, LastName FROM Employees;
- INSERT: Adds new data to the database.
INSERT INTO Employees (EmployeeID, FirstName, LastName, BirthDate)
VALUES (1, 'John', 'Doe', '1980-01-01');
- UPDATE: Modifies existing data.
UPDATE Employees
SET Email = '[email protected]'
WHERE EmployeeID = 1;
- DELETE: Removes data from the database.
DELETE FROM Employees
WHERE EmployeeID = 1;
Data Control Language (DCL)
DCL commands control access to data in the database, primarily through:
- GRANT: Gives user’s access privileges.
GRANT SELECT ON Employees TO user;
- REVOKE: Removes user’s access privileges.
REVOKE SELECT ON Employees FROM user;
Transaction Control Language (TCL)
TCL commands manage changes made by DML commands and ensure data integrity:
- COMMIT: Saves all changes made during the current transaction.
COMMIT;
ROLLBACK: Reverts all changes made during the current transaction.
ROLLBACK;
SAVEPOINT: Sets a savepoint within a transaction.
SAVEPOINT SavePoint1;
Advanced SQL Features
Joins
Joins are used to combine rows from two or more tables based on related columns.
- INNER JOIN: Returns rows when there is a match in both tables.
SELECT Employees.FirstName, Departments.DepartmentName
FROM Employees
INNER JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID;
LEFT JOIN: Returns all rows from the left table, and the matched rows from the right table.
SELECT Employees.FirstName, Departments.DepartmentName
FROM Employees
LEFT JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID;
RIGHT JOIN: Returns all rows from the right table, and the matched rows from the left table.
SELECT Employees.FirstName, Departments.DepartmentName
FROM Employees
RIGHT JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID;
FULL OUTER JOIN: Returns rows when there is a match in one of the tables.
SELECT Employees.FirstName, Departments.DepartmentName
FROM Employees
FULL OUTER JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID;
Subqueries
A subquery is a query nested inside another query, allowing for more complex retrieval of data.
- Example:
SELECT FirstName, LastName
FROM Employees
WHERE EmployeeID IN (SELECT EmployeeID FROM Sales WHERE Amount > 10000);
Indexes
Indexes improve the speed of data retrieval operations on a database table.
Creating an Index:
CREATE INDEX idx_lastname ON Employees (LastName);
Views
A view is a virtual table based on the result of a SELECT query.
- Creating a View:
CREATE VIEW EmployeeView AS
SELECT FirstName, LastName, DepartmentName
FROM Employees
JOIN Departments ON Employees.DepartmentID = Departments.DepartmentID;
The Importance of SQL in Modern Business
Data Management
SQL is essential for managing large volumes of data efficiently. It allows businesses to store, retrieve, and manipulate data seamlessly, ensuring data integrity and security.
Decision Making
SQL enables data-driven decision-making by providing tools to query and analyze data. Businesses can derive insights, identify trends, and make informed decisions based on accurate and timely data.
Scalability
SQL databases can handle large-scale applications with numerous transactions and users. They offer scalability and reliability, making them suitable for enterprises of all sizes.
Interoperability
SQL is widely supported across various database systems, including MySQL, PostgreSQL, Oracle, and SQL Server. This interoperability ensures that SQL skills are transferable and applicable across different platforms.
Best Practices for Using SQL
Write Clear and Efficient Queries
- Use Proper Formatting: Indent and align your SQL statements for readability.
- **Avoid SELECT ***: Specify only the columns you need.
- Use Joins Efficiently: Be mindful of the performance impact of joins.
- Indexing: Index columns that are frequently used in WHERE clauses.
Ensure Data Security
- Use Parameterized Queries: Prevent SQL injection attacks by using parameterized queries.
- Access Control: Implement proper access controls using GRANT and REVOKE statements.
- Data Encryption: Encrypt sensitive data at rest and in transit.
Maintain Data Integrity
- Use Constraints: Enforce data integrity with primary keys, foreign keys, and unique constraints.
- Transaction Management: Use transactions to ensure data consistency.
Regular Maintenance
- Backup and Recovery: Implement regular backup and recovery procedures.
- Performance Tuning: Monitor and optimize database performance regularly.
Future Trends in SQL
Integration with Big Data Technologies
SQL is increasingly being integrated with big data technologies such as Hadoop and Spark, enabling seamless querying and analysis of large datasets.
SQL and NoSQL Hybrid Solutions
The line between SQL and NoSQL databases is blurring, with hybrid solutions offering the best of both worlds. This trend allows for more flexible and scalable database architectures.
Machine Learning Integration
SQL is being integrated with machine learning frameworks, allowing for advanced data analysis and predictive modeling directly within the database.
Cloud-Based SQL Solutions
Cloud-based SQL databases are becoming more popular, offering scalability, flexibility, and cost-efficiency. Services like Amazon RDS, Google Cloud SQL, and Microsoft Azure SQL Database are leading this trend.
The Complete PL/SQL Bootcamp : Advanced PL/SQL| Course.
Conclusion
Structured Query Language (SQL) remains a vital tool in the realm of data management and analysis. Its robust set of features, coupled with its ease of use and flexibility, make it indispensable for businesses and individuals alike. As data continues to grow in importance, SQL will remain a cornerstone of database management, evolving to meet the demands of modern data-driven environments. Understanding and mastering SQL is essential for anyone looking to excel in the fields of data science, software development, and beyond.