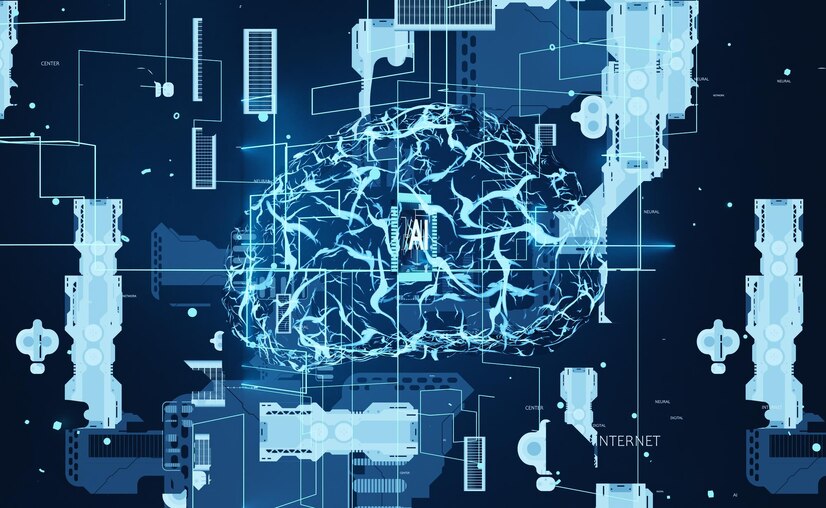
Transformer neural networks have revolutionized the field of natural language processing (NLP) and machine learning. Originally introduced by Vaswani et al. in their seminal paper “Attention is All You Need,” transformers have since become the backbone of many state-of-the-art models, including BERT, GPT, and T5. In this comprehensive guide, we will explore how to program transformer neural networks using PyTorch, one of the most popular deep learning frameworks.
Introduction to Transformer Neural Networks
Before diving into the code, it’s essential to understand the core concepts of transformer neural networks. Unlike traditional recurrent neural networks (RNNs) that process data sequentially, transformers process data in parallel, making them highly efficient and scalable. The key innovation behind transformers is the self-attention mechanism, which allows the model to focus on different parts of the input sequence with varying importance.
Why Use Transformers?
Transformers have several advantages over traditional RNNs and convolutional neural networks (CNNs), particularly in handling sequential data. Some of the key benefits include:
- Parallelization: Unlike RNNs, transformers process entire sequences at once, allowing for better utilization of GPUs and faster training times.
- Long-Range Dependencies: Transformers can capture long-range dependencies in the data, making them ideal for tasks like language translation, text summarization, and sentiment analysis.
- Scalability: Transformers can easily scale to handle very large datasets, which is crucial for training large language models.
Getting Started with PyTorch
PyTorch is a flexible and intuitive deep learning framework that has gained widespread adoption in the research and developer community. Before we begin programming transformers, ensure that you have PyTorch installed. You can install it using pip:
Additionally, we’ll need the Hugging Face transformers
library, which provides pre-trained models and utilities to work with transformers:
pip install transformers
Building a Transformer Model from Scratch
While the Hugging Face library provides pre-built transformer models, it’s crucial to understand how to build one from scratch to gain deeper insights into how transformers work. In this section, we’ll implement a basic transformer model using PyTorch.
1. The Self-Attention Mechanism
The self-attention mechanism is the heart of the transformer model. It allows the model to weigh the importance of different words in a sentence when making predictions. Here’s how you can implement the scaled dot-product attention mechanism in PyTorch:
import torch
import torch.nn as nn
class ScaledDotProductAttention(nn.Module):
def __init__(self, d_k):
super(ScaledDotProductAttention, self).__init__()
self.d_k = d_k
def forward(self, query, key, value, mask=None):
scores = torch.matmul(query, key.transpose(-2, -1)) / torch.sqrt(self.d_k)
if mask is not None:
scores = scores.masked_fill(mask == 0, -1e9)
attention = torch.nn.functional.softmax(scores, dim=-1)
output = torch.matmul(attention, value)
return output, attention
2. Multi-Head Attention
Transformers use multiple self-attention mechanisms, known as multi-head attention, to capture different aspects of the input data. Here’s how to implement it:
class MultiHeadAttention(nn.Module):
def __init__(self, d_model, num_heads):
super(MultiHeadAttention, self).__init__()
self.num_heads = num_heads
self.d_k = d_model // num_heads
self.query_linear = nn.Linear(d_model, d_model)
self.key_linear = nn.Linear(d_model, d_model)
self.value_linear = nn.Linear(d_model, d_model)
self.out_linear = nn.Linear(d_model, d_model)
self.attention = ScaledDotProductAttention(self.d_k)
def forward(self, query, key, value, mask=None):
batch_size = query.size(0)
# Linear projections
query = self.query_linear(query).view(batch_size, -1, self.num_heads, self.d_k)
key = self.key_linear(key).view(batch_size, -1, self.num_heads, self.d_k)
value = self.value_linear(value).view(batch_size, -1, self.num_heads, self.d_k)
# Apply attention on all the projected vectors
output, attention = self.attention(query, key, value, mask)
# Concatenate the heads and apply the final linear layer
output = output.view(batch_size, -1, self.num_heads * self.d_k)
output = self.out_linear(output)
return output, attention
3. Position-Wise Feed-Forward Networks
After the multi-head attention mechanism, the transformer applies a position-wise feed-forward network (FFN) to each position separately and identically:
class PositionwiseFeedForward(nn.Module):
def __init__(self, d_model, d_ff):
super(PositionwiseFeedForward, self).__init__()
self.fc1 = nn.Linear(d_model, d_ff)
self.fc2 = nn.Linear(d_ff, d_model)
self.relu = nn.ReLU()
def forward(self, x):
return self.fc2(self.relu(self.fc1(x)))
4. Positional Encoding
Transformers do not have recurrence or convolution, so they use positional encodings to inject information about the relative or absolute position of tokens in the sequence:
class PositionalEncoding(nn.Module):
def __init__(self, d_model, max_len=5000):
super(PositionalEncoding, self).__init__()
pe = torch.zeros(max_len, d_model)
position = torch.arange(0, max_len, dtype=torch.float).unsqueeze(1)
div_term = torch.exp(torch.arange(0, d_model, 2).float() * (-torch.log(torch.tensor(10000.0)) / d_model))
pe[:, 0::2] = torch.sin(position * div_term)
pe[:, 1::2] = torch.cos(position * div_term)
pe = pe.unsqueeze(0).transpose(0, 1)
self.register_buffer('pe', pe)
def forward(self, x):
return x + self.pe[:x.size(0), :]
5. Transformer Encoder Layer
The transformer encoder layer combines the multi-head attention mechanism and the position-wise feed-forward network:
class TransformerEncoderLayer(nn.Module):
def __init__(self, d_model, num_heads, d_ff):
super(TransformerEncoderLayer, self).__init__()
self.self_attn = MultiHeadAttention(d_model, num_heads)
self.ffn = PositionwiseFeedForward(d_model, d_ff)
self.norm1 = nn.LayerNorm(d_model)
self.norm2 = nn.LayerNorm(d_model)
def forward(self, x, mask=None):
attn_output, _ = self.self_attn(x, x, x, mask)
x = self.norm1(x + attn_output)
ffn_output = self.ffn(x)
x = self.norm2(x + ffn_output)
return x
6. Transformer Model
Finally, the complete transformer model can be constructed by stacking multiple encoder layers:
class TransformerEncoder(nn.Module):
def __init__(self, num_layers, d_model, num_heads, d_ff, input_dim, max_len):
super(TransformerEncoder, self).__init__()
self.positional_encoding = PositionalEncoding(d_model, max_len)
self.layers = nn.ModuleList([TransformerEncoderLayer(d_model, num_heads, d_ff) for _ in range(num_layers)])
self.embedding = nn.Embedding(input_dim, d_model)
def forward(self, x, mask=None):
x = self.embedding(x)
x = self.positional_encoding(x)
for layer in self.layers:
x = layer(x, mask)
return x
Fine-Tuning Pre-Trained Transformer Models
While building transformers from scratch is educational, many practical applications benefit from fine-tuning pre-trained models. The Hugging Face transformers
library provides an easy way to fine-tune models like BERT or GPT-2 for specific tasks.
1. Loading Pre-Trained Models
You can load a pre-trained transformer model and tokenizer as follows:
from transformers import BertTokenizer, BertForSequenceClassification
tokenizer = BertTokenizer.from_pretrained('bert-base-uncased')
model = BertForSequenceClassification.from_pretrained('bert-base-uncased')
2. Tokenizing Input Data
Next, tokenize your input data to prepare it for the model:
inputs = tokenizer("Hello, this is a sample input.", return_tensors="pt")
labels = torch.tensor([1]).unsqueeze(0) # Batch size 1
outputs = model(**inputs, labels=labels)
loss = outputs.loss
logits = outputs.logits
3. Fine-Tuning
You can fine-tune the model using your dataset and an optimizer like AdamW:
from transformers import AdamW
optimizer = AdamW(model.parameters(), lr=1e-5)
loss.backward()
optimizer.step()
Practical Applications of Transformer Models
Transformers have transformed various domains of AI, particularly in NLP. Here are a few applications:
1. Text Classification
Transformers can classify text into predefined categories. For instance, you can use BERT to classify tweets as positive, negative, or neutral.
2. Machine Translation
Models like GPT-2 can be fine-tuned for translating text from one language to another.
3. Text Summarization
Using transformers, you can generate summaries of long documents, making them useful in content creation and news aggregation.
4. Question Answering
Transformers can be used to build systems that automatically answer questions based on a given context, similar to how search engines provide quick answers.
Conclusion
Programming transformer neural networks with PyTorch provides a powerful toolset for tackling complex AI problems. Whether building a transformer from scratch or fine-tuning a pre-trained model, PyTorch offers the flexibility and performance needed for cutting-edge research and development. As transformers continue to dominate NLP and other AI fields, mastering their implementation will open up numerous opportunities for innovation and application.
By following this comprehensive guide, you should now have a solid understanding of how to program transformers using PyTorch. With this knowledge, you can confidently build and fine-tune transformer models for your specific tasks.