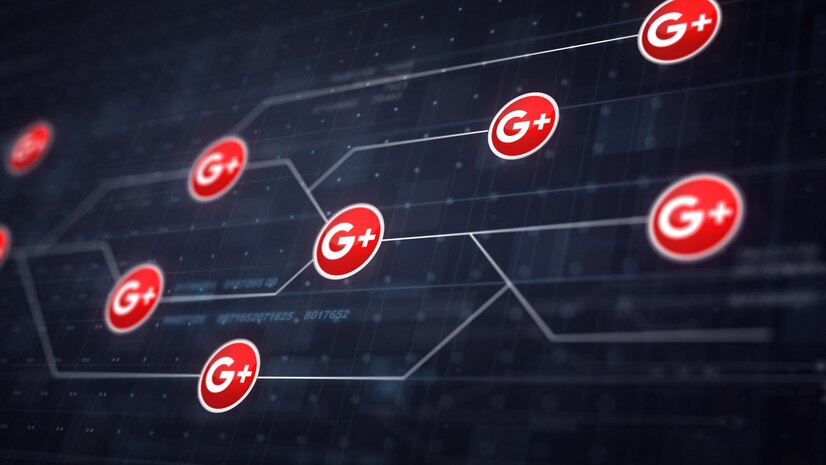
Introduction:
Arbitrage trading remains one of the most compelling strategies in the financial markets, offering the potential to generate profits with minimal risk. Among the various arbitrage techniques, triangular arbitrage is particularly intriguing. This strategy involves exploiting price differences between three different assets to secure a profit. When applied to the cryptocurrency markets, both centralized exchanges (CEXs) and decentralized exchanges (DEXs) offer unique opportunities for triangular arbitrage. This article will guide you through the process of developing a triangular arbitrage algorithm that can be deployed across both CEXs and DEXs using Python.
Understanding Triangular Arbitrage
Triangular arbitrage is a strategy that capitalizes on discrepancies between three currencies. The concept is straightforward: a trader exchanges one currency for a second, the second currency for a third, and then the third currency back to the first, ideally ending up with more of the original currency than they started with.
Example in the Crypto Market: Let’s consider three cryptocurrencies: BTC, ETH, and USDT. A triangular arbitrage opportunity might involve converting BTC to ETH, ETH to USDT, and finally, USDT back to BTC. If the combined exchange rates across these transactions result in more BTC than the original amount, a profit is realized.
Key Components of a Triangular Arbitrage Algorithm
To develop an effective triangular arbitrage algorithm, certain key components must be implemented:
- Market Data Collection:
- Accurate and real-time market data from various exchanges is essential. Use APIs provided by centralized and decentralized exchanges to fetch current price quotes.
- Centralized exchanges like Binance, Coinbase, and Kraken offer robust APIs for fetching market data.
- Decentralized exchanges, on the other hand, typically interact with smart contracts on blockchains like Ethereum and Binance Smart Chain (BSC). Tools like Web3.py can be used to interface with DEXs like Uniswap or PancakeSwap.
- Price Discrepancy Detection:
- The algorithm needs to continuously monitor the exchange rates between the three assets across different exchanges.
- Identify when the product of the exchange rates deviates from 1, indicating a potential arbitrage opportunity.
- Transaction Execution:
- For centralized exchanges, executing trades involves using the exchange’s API to place buy and sell orders.
- In decentralized exchanges, transactions occur directly on the blockchain. Here, smart contracts and liquidity pools are involved, so the algorithm must be able to interact with these contracts.
- Profit Calculation and Risk Management:
- Before executing trades, the algorithm should calculate the potential profit, considering transaction fees, slippage, and network delays.
- Implement risk management strategies to avoid losses, such as setting stop-loss levels or limiting the capital allocated to any single trade.
Developing the Algorithm in Python
Python offers a versatile environment for developing and deploying trading algorithms. Below is a simplified approach to building a triangular arbitrage algorithm:
Step 1: Setting Up the Environment
import ccxt # for centralized exchange interaction
from web3 import Web3 # for decentralized exchange interaction
import time
# Connect to exchanges
binance = ccxt.binance()
uniswap = Web3(Web3.HTTPProvider('<Infura or other Ethereum node URL>'))
Step 2: Fetching Market Data
# Fetching data from Binance (Centralized)
symbol1 = 'BTC/USDT'
symbol2 = 'ETH/BTC'
symbol3 = 'ETH/USDT'
order_book1 = binance.fetch_order_book(symbol1)
order_book2 = binance.fetch_order_book(symbol2)
order_book3 = binance.fetch_order_book(symbol3)
# Fetching data from Uniswap (Decentralized)
def get_uniswap_price(pair_address):
contract = uniswap.eth.contract(address=pair_address, abi=uniswap_abi)
reserves = contract.functions.getReserves().call()
return reserves[0] / reserves[1] # Example calculation
Step 3: Identifying Arbitrage Opportunities
# Calculate potential arbitrage profits
rate1 = order_book1['bids'][0][0]
rate2 = order_book2['asks'][0][0]
rate3 = order_book3['bids'][0][0]
arbitrage_opportunity = rate1 * rate2 * rate3
if arbitrage_opportunity > 1.01: # Threshold to cover fees
print("Arbitrage opportunity detected!")
Step 4: Executing Trades
# Centralized exchange execution
amount = 0.1 # example amount
binance.create_order(symbol1, 'market', 'buy', amount)
binance.create_order(symbol2, 'market', 'sell', amount)
binance.create_order(symbol3, 'market', 'buy', amount)
# Decentralized exchange execution
tx = uniswap.eth.contract(address=pair_address, abi=uniswap_abi).functions.swapExactTokensForTokens(
amount, min_out, [token1, token2, token3], wallet_address, deadline).buildTransaction()
Step 5: Implementing Risk Management
# Check profits
initial_balance = 1 # example balance in BTC
final_balance = arbitrage_opportunity * initial_balance
if final_balance <= initial_balance:
print("No profit. Skipping trade.")
else:
print(f"Profit: {final_balance - initial_balance} BTC")
Challenges and Considerations
- Slippage and Fees:
- Slippage can occur when the market price moves during the time it takes to execute trades. This is particularly problematic on DEXs due to the variable liquidity in pools.
- Always account for transaction fees on both CEXs and DEXs. In the case of DEXs, gas fees on blockchains like Ethereum can be substantial, potentially erasing profits.
- Execution Speed:
- Timing is critical in arbitrage strategies. Ensure your algorithm can execute trades as quickly as possible to lock in the price discrepancies before they disappear.
- On DEXs, transaction times can vary depending on network congestion, which adds an additional layer of complexity.
- Regulatory Compliance:
- Ensure that your trading activities comply with the regulations of the jurisdictions in which you operate. This includes adhering to any KYC/AML requirements on CEXs and understanding the legal implications of smart contract interactions on DEXs.
Triangular Arbitrage for Crypto with Python – Course.
Conclusion
Building a triangular arbitrage algorithm for both centralized and decentralized exchanges offers the potential for consistent profits by exploiting price discrepancies in the cryptocurrency markets. By carefully developing and testing your algorithm using Python, you can create a robust tool that navigates the complexities of both CEXs and DEXs. However, it is crucial to consider the various challenges, such as slippage, fees, and execution speed, to ensure the algorithm’s effectiveness and profitability. Continuous monitoring, optimization, and risk management will be key to long-term success in the ever-evolving crypto trading landscape.