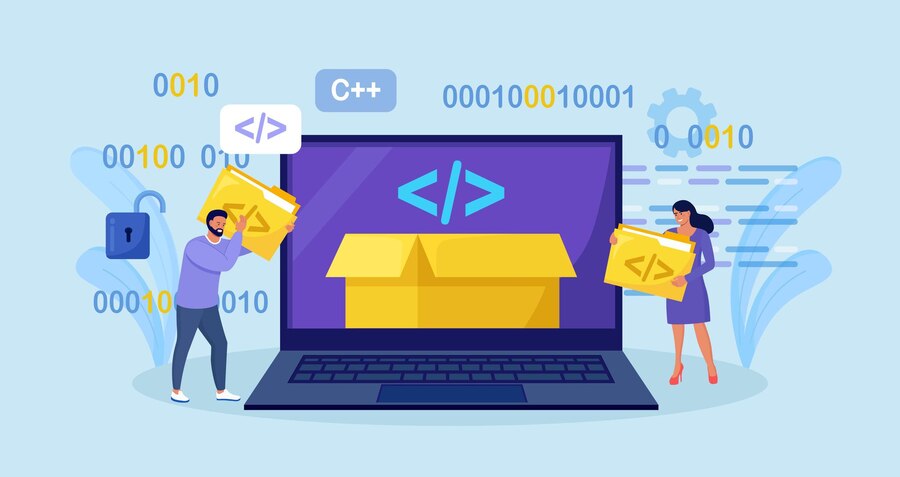
Introduction:
In the rapidly evolving world of cryptocurrency, automated trading bots have become a powerful tool for traders looking to capitalize on market inefficiencies. One of the most popular strategies employed by these bots is statistical arbitrage. This article will guide you through the process of building a statistical arbitrage bot in the crypto market using Python.
Understanding Statistical Arbitrage in Crypto
Statistical arbitrage, often abbreviated as stat arb, is a trading strategy that exploits the price differences of similar or correlated assets. Unlike traditional arbitrage, which focuses on buying and selling the same asset across different exchanges, statistical arbitrage looks for opportunities across a basket of assets. In the context of crypto.
Why Choose Statistical Arbitrage?
Statistical arbitrage is particularly well-suited for the crypto market because of its inherent volatility and the vast number of tradable assets. The strategy relies on mathematical models to predict the mean-reversion of prices, making it a data-driven approach that minimizes the influence of emotional trading. Additionally, the high-frequency nature of the crypto market provides ample opportunities for executing stat arb strategies.
Setting Up Your Python Environment
Before diving into the code, it’s essential to set up your Python environment with the necessary libraries. Python’s versatility and extensive ecosystem make it an ideal choice for developing trading bots. Here’s a list of the key libraries you’ll need:
- Pandas: For data manipulation and analysis.
- NumPy: For numerical computations.
- Matplotlib: For data visualization.
- Scikit-learn: For machine learning models.
- CCXT: For interacting with cryptocurrency exchanges.
To install these libraries, you can use pip, Python’s package installer:
pip install pandas numpy matplotlib scikit-learn ccxt
Data Collection and Preprocessing
The first step in building your statistical arbitrage bot is collecting historical price data for the cryptocurrencies you plan to trade. You can use the CCXT library to pull this data from various exchanges. Here’s an example of how to collect data using CCXT:
import ccxt
import pandas as pd
exchange = ccxt.binance()
symbol = 'BTC/USDT'
timeframe = '1h'
since = exchange.parse8601('2022-01-01T00:00:00Z')
# Fetching historical data
data = exchange.fetch_ohlcv(symbol, timeframe, since)
df = pd.DataFrame(data, columns=['timestamp', 'open', 'high', 'low', 'close', 'volume'])
df['timestamp'] = pd.to_datetime(df['timestamp'], unit='ms')
After collecting the data, you’ll need to preprocess it by normalizing prices, handling missing data, and calculating key indicators that your bot will use to make trading decisions.
Building the Statistical Model
The core of your arbitrage bot lies in the statistical model that identifies trading opportunities. A common approach is to use a pairs trading strategy, which involves selecting two cryptocurrencies with historically correlated price movements. The idea is to trade when the price spread between the two assets diverges from its historical mean and to close the trade when the spread reverts to the mean.
Here’s a basic example of how to implement a pairs trading strategy:
import numpy as np
# Calculating the price spread
df['spread'] = df['close_1'] - df['close_2']
# Calculating the mean and standard deviation of the spread
mean_spread = df['spread'].mean()
std_spread = df['spread'].std()
# Defining entry and exit points
df['signal'] = np.where(df['spread'] > mean_spread + std_spread, -1, np.nan)
df['signal'] = np.where(df['spread'] < mean_spread - std_spread, 1, df['signal'])
df['signal'] = df['signal'].fillna(0)
In this code snippet, the bot generates a signal to buy or sell based on the price spread between two assets. When the spread is significantly higher than the mean, the bot goes short (sells); when it’s significantly lower, the bot goes long (buys).
Backtesting Your Strategy
Before deploying your bot in a live environment, it’s crucial to backtest your strategy using historical data. Backtesting allows you to simulate trades and assess the profitability of your strategy under different market conditions. Here’s how you can backtest your pairs trading strategy:
# Calculating returns
df['returns'] = df['signal'].shift(1) * df['spread'].pct_change()
# Calculating cumulative returns
df['cumulative_returns'] = (df['returns'] + 1).cumprod()
# Plotting the results
df['cumulative_returns'].plot()
By analyzing the cumulative returns, you can determine whether your strategy would have been profitable in the past. It’s important to optimize your model by tweaking parameters such as the lookback period for calculating the mean spread.
Deploying the Bot in a Live Environment
Once you’re confident in your backtesting results, it’s time to deploy your bot in a live trading environment. This involves connecting your bot to a crypto exchange via API and setting up mechanisms to execute trades automatically. It’s essential to start with small trade sizes and monitor the bot closely to ensure it behaves as expected in real market conditions.
# Example of placing a trade
order = exchange.create_market_order(symbol, 'buy', amount)
Statistical Arbitrage Bot Build in Crypto with Python (A-Z)| Course.
Risk Management and Optimization
Risk management is a critical aspect of any trading strategy. Implementing stop-loss orders, position sizing rules, and diversification can help mitigate potential losses. Additionally, continuously optimizing your strategy by analyzing performance metrics and adapting to changing market conditions is essential for long-term success.
Conclusion
Building a statistical arbitrage bot in crypto using Python offers a unique opportunity to capitalize on market inefficiencies in the rapidly growing cryptocurrency space. By following this guide, you can create a robust bot that leverages statistical models to identify and execute profitable trades. Remember, successful trading requires continuous learning.