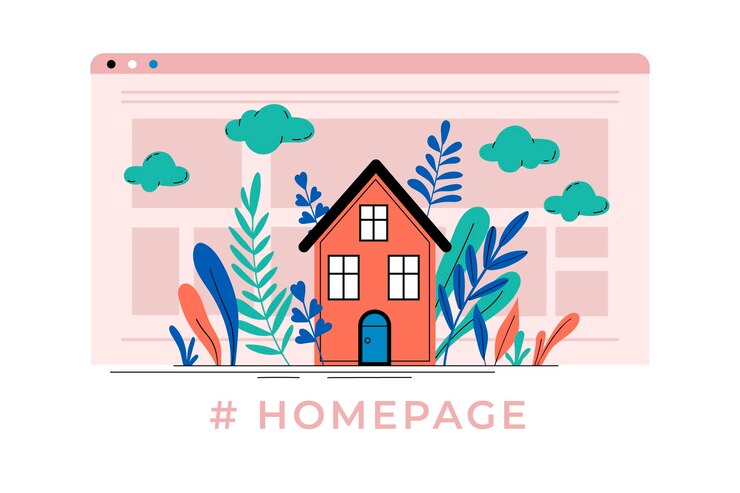
Introduction:
Creating a personal website is a fantastic way to showcase your hometown, share your experiences, and highlight what makes your community unique. HTML (HyperText Markup Language) and CSS (Cascading Style Sheets) are the fundamental building blocks of the web and offer a powerful combination for building visually appealing and functional websites. This guide will walk you through the process of building a “Hometown Homepage” using HTML and CSS, whether you’re a beginner or someone looking to sharpen your web development skills.
Why Build a Hometown Homepage?
A hometown homepage is a personalized website dedicated to your place of origin. It could serve multiple purposes, such as:
- Showcasing Local Culture: Highlighting the traditions, festivals, and cultural aspects that make your hometown unique.
- Promoting Tourism: Providing information for potential visitors, including local attractions, events, and accommodations.
- Preserving Memories: Documenting personal experiences and memories associated with your hometown.
- Networking: Connecting with other individuals from your hometown or those interested in learning more about it.
Building a hometown homepage can also be a fun and educational project that hones your skills in HTML and CSS, two of the most essential web technologies.
Build Responsive Real-World Websites| Course
Getting Started: The Basics of HTML and CSS
Before diving into the creation of your homepage, it’s important to understand the basics of HTML and CSS:
HTML (HyperText Markup Language)
HTML is the standard language for creating web pages. It structures the content on the web by using elements (tags) to define headings, paragraphs, links, images, and other types of content. Here’s a simple example of HTML code:
<!DOCTYPE html>
<html>
<head>
<title>My Hometown</title>
</head>
<body>
<h1>Welcome to My Hometown</h1>
<p>This is a brief introduction to my hometown, where I grew up and made countless memories.</p>
</body>
</html>
In this example:
<!DOCTYPE html>
declares the document type.<html>
is the root element that contains all other HTML elements.<head>
includes meta-information like the page title.<body>
contains the main content of the webpage.
CSS (Cascading Style Sheets)
CSS is used to control the presentation of the webpage. It allows you to apply styles to HTML elements, such as colors, fonts, layouts, and spacing. Here’s an example of CSS code:
body {
font-family: Arial, sans-serif;
background-color: #f4f4f4;
color: #333;
}
h1 {
color: #0073e6;
text-align: center;
}
In this example:
body
applies styles to the entire webpage, such as the font and background color.h1
targets the heading element to change its color and center its alignment.
Now that you have a basic understanding of HTML and CSS, let’s move on to building your hometown homepage.
Step 1: Planning Your Hometown Homepage
Before you start coding, it’s essential to plan your homepage. Consider the following aspects:
Content
What content do you want to include on your homepage? Here are some ideas:
- Introduction: A brief introduction to your hometown, its history, and what makes it special.
- Local Attractions: Highlight popular tourist spots, parks, museums, and historical landmarks.
- Events and Festivals: List annual events, festivals, and celebrations unique to your hometown.
- Gallery: Include images or a photo gallery showcasing the beauty and life of your hometown.
- Contact Information: Provide ways for visitors to get in touch with you or local authorities.
Layout
Consider how you want to organize your content. A simple layout might include:
- Header: The top section with the site title and navigation links.
- Main Content Area: The central section containing text, images, and other media.
- Sidebar: A section for additional information, such as links to social media or related articles.
- Footer: The bottom section with contact information or copyright details.
Design
Think about the visual style of your homepage. What colors, fonts, and images best represent your hometown? You can also consider the mood you want to convey—whether it’s vibrant and lively or calm and serene.
Step 2: Creating the HTML Structure
With your plan in mind, let’s start coding the HTML structure of your homepage.
HTML Document Setup
Create a new file named index.html
and add the basic structure:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Welcome to [Your Hometown]</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<header>
<h1>Welcome to [Your Hometown]</h1>
<nav>
<ul>
<li><a href="#about">About</a></li>
<li><a href="#attractions">Attractions</a></li>
<li><a href="#events">Events</a></li>
<li><a href="#contact">Contact</a></li>
</ul>
</nav>
</header>
<main>
<section id="about">
<h2>About [Your Hometown]</h2>
<p>[Your Hometown] is known for its rich history and vibrant culture...</p>
</section>
<section id="attractions">
<h2>Local Attractions</h2>
<ul>
<li>[Attraction 1]</li>
<li>[Attraction 2]</li>
<li>[Attraction 3]</li>
</ul>
</section>
<section id="events">
<h2>Events and Festivals</h2>
<p>Join us for the annual [Festival Name] held every [Month]...</p>
</section>
</main>
<footer>
<p>Contact us at [Email Address].</p>
</footer>
</body>
</html>
This structure includes:
- A header with a navigation menu.
- Three main sections: About, Attractions, and Events.
- A footer with contact information.
Replace the placeholder text (e.g., [Your Hometown]
) with actual content related to your hometown.
Step 3: Styling Your Homepage with CSS
Next, create a styles.css
file to style your homepage. Here’s an example:
/* General Styles */
body {
font-family: 'Segoe UI', Tahoma, Geneva, Verdana, sans-serif;
background-color: #ffffff;
color: #333333;
margin: 0;
padding: 0;
}
header {
background-color: #0073e6;
color: #ffffff;
padding: 10px 0;
text-align: center;
}
nav ul {
list-style-type: none;
padding: 0;
}
nav ul li {
display: inline;
margin: 0 10px;
}
nav ul li a {
color: #ffffff;
text-decoration: none;
}
main {
padding: 20px;
}
section {
margin-bottom: 20px;
}
h2 {
color: #0073e6;
}
footer {
background-color: #f4f4f4;
color: #333333;
text-align: center;
padding: 10px 0;
position: fixed;
width: 100%;
bottom: 0;
}
This CSS will:
- Set a clean, simple font for the entire site.
- Style the header with a blue background and white text.
- Arrange the navigation menu items horizontally.
- Add padding to the main content area for readability.
- Style the section headings and footer.
You can customize the colors, fonts, and layout to better match the theme of your hometown.
Step 4: Enhancing Your Homepage with Images and Media
Visual content is key to making your hometown homepage engaging. Here’s how you can add images and media:
Adding Images
To add images, use the <img>
tag in HTML:
<section id="gallery">
<h2>Gallery</h2>
<img src="images/attraction1.jpg" alt="[Attraction 1]" width="300">
<img src="images/attraction2.jpg" alt="[Attraction 2]" width="300">
<img src="images/attraction3.jpg" alt="[Attraction 3]" width="300">
</section>
Ensure you replace src
with the actual path to your image files. The alt
attribute provides alternative text for screen readers, enhancing accessibility.
Embedding Videos
You can also embed videos using the <video>
tag or by embedding YouTube videos:
<section id="video">
<h2>Explore [Your Hometown]</h2>
<video controls>
<source src="videos/hometown.mp4" type="video/mp4">
Your browser does not support the video tag.
</video>
</section>
<!-- OR -->
<section id="video">
<h2>Explore [Your Hometown]</h2>
<iframe width="560" height="315" src="https://www.youtube.com/embed/your_video_id" frameborder="0" allow="accelerometer; autoplay; encrypted-media; gyroscope; picture-in-picture" allowfullscreen></iframe>
</section>